On this article of Java Design Sample, we are going to have a look at the Flyweight Design Sample. It is without doubt one of the Structural Design Patterns. We’ll study what this sample is all about. After that, we’ll have a look at the sample’s design benefits, utilization, and drawbacks.
The flyweight design sample is utilized when we have to assemble a lot of objects belonging to a category. Since every object requires reminiscence, the flyweight design sample can be utilized to share objects and reduce the demand for reminiscence in low-memory gadgets like cell gadgets or embedded methods. In essence, a flyweight object has two forms of traits: intrinsic and extrinsic.
The flyweight object shops and shares an intrinsic state property that isn’t depending on the context of the flyweight. We must always make intrinsic states immutable as the most effective apply. Extrinsic states can’t be transmitted since they rely upon the context of the flyweight. Consumer objects should present the flyweight object with the extrinsic state when creating the item as a result of they keep it.
We should separate Object attributes into intrinsic and extrinsic qualities to use the flyweight sample. Extrinsic attributes are set by shopper code and are used to hold out particular duties, whereas intrinsic properties make an object distinctive. An Object Circle, for example, might have exterior traits like shade and breadth.
We should construct a Flyweight manufacturing unit that outputs shared objects to use the flyweight sample. Let’s think about, for the sake of our instance, that we have to draw one thing with traces and ovals. In consequence, we could have a Form interface and its precise implementations, Line and Oval. In distinction to Line, the oval class has an intrinsic attribute that determines whether or not or to not fill the Oval with a selected shade.
The flyweight design sample is used:
Within the aforementioned instance, we’re making a Paint Brush software the place the client might select from three totally different brush sorts: THICK, THIN, AND MEDIUM. Solely the content material shade will alter between every thick (or skinny or medium) brush’s drawings of the content material.Let’s see what are the steps to implement the flyweight design sample in Java.
Step1: First, let’s create an interface Brush to attract the work:
Step 2: Subsequent, let’s create
Step 3: Subsequent, let’s create implementing lessons
ThinBrush.java
MediumBrush.java
Right here, the shopper will provide the extrinsic attribute of brush shade; else, the comb could have no variations. Subsequently, in essence, we are going to solely produce a sure measurement of the comb when the colour is totally different. We are going to reuse it when a unique shopper or scenario requires that brush measurement and shade.
Step 4: Subsequent, let’s Create the manufacturing unit
Step 5: Subsequent, let’s create the shopper program
Right here, as you possibly can see the
Flyweight Design Sample
Let’s check out among the actual world instance of the Flyweight Design Sample.
We are able to hold only one merchandise within the system due to the singleton design Sample. In different phrases, as soon as the mandatory object is produced, we’re unable to provide any extra. The present object should be used all through your complete software. When it’s vital to construct a lot of objects which can be comparable however differ primarily based on an extrinsic attribute equipped by the shopper, the flyweight sample is utilized.
On this submit, we talked concerning the Flyweight Design Sample. We noticed the real-world examples together with what are some benefits and drawbacks of utilizing this sample. We additionally noticed a Java implementation for this design sample. You possibly can all the time examine our GitHub repository for the most recent supply code.
The ValueOf() strategies in all wrapper lessons make use of cached objects, demonstrating the applying of the Flyweight design precept. The Java String class’s implementation of the String Pool is the most effective case research.
Flyweight Design Sample
The flyweight design sample is utilized when we have to assemble a lot of objects belonging to a category. Since every object requires reminiscence, the flyweight design sample can be utilized to share objects and reduce the demand for reminiscence in low-memory gadgets like cell gadgets or embedded methods. In essence, a flyweight object has two forms of traits: intrinsic and extrinsic.
The flyweight object shops and shares an intrinsic state property that isn’t depending on the context of the flyweight. We must always make intrinsic states immutable as the most effective apply. Extrinsic states can’t be transmitted since they rely upon the context of the flyweight. Consumer objects should present the flyweight object with the extrinsic state when creating the item as a result of they keep it.
We should separate Object attributes into intrinsic and extrinsic qualities to use the flyweight sample. Extrinsic attributes are set by shopper code and are used to hold out particular duties, whereas intrinsic properties make an object distinctive. An Object Circle, for example, might have exterior traits like shade and breadth.
We should construct a Flyweight manufacturing unit that outputs shared objects to use the flyweight sample. Let’s think about, for the sake of our instance, that we have to draw one thing with traces and ovals. In consequence, we could have a Form interface and its precise implementations, Line and Oval. In distinction to Line, the oval class has an intrinsic attribute that determines whether or not or to not fill the Oval with a selected shade.
1. When to make use of Flyweight Design Sample
The flyweight design sample is used:
- When the applying ought to create a lot of objects.
- When the method of making an object would possibly take numerous time and requires numerous reminiscence.
- When the extrinsic attributes of an Object must be outlined by the shopper software program. The properties of an object may be separated into intrinsic and extrinsic properties.
2. Flyweight Sample utilizing Java
Within the aforementioned instance, we’re making a Paint Brush software the place the client might select from three totally different brush sorts: THICK, THIN, AND MEDIUM. Solely the content material shade will alter between every thick (or skinny or medium) brush’s drawings of the content material.Let’s see what are the steps to implement the flyweight design sample in Java.
Step1: First, let’s create an interface Brush to attract the work:
Code:
public interface Brush {
public void setColor(String shade);
public void draw(String content material);
}
Step 2: Subsequent, let’s create
ENUM
BrushSize for brush sizes.
Code:
public enum BrushSize {
THIN,
MEDIUM,
THICK
}
Step 3: Subsequent, let’s create implementing lessons
ThickBrush
, ThinBrush
, and MediumBrush
. They are going to implement the Brush
interface.
Code:
public class ThickBrush implements Brush {
/*
intrinsic state - shareable
*/
last BrushSize brushSize = BrushSize.THICK;
/*
extrinsic state - equipped by the shopper
*/
personal String shade = null;
public void setColor(String shade) {
this.shade = shade;
}
@Override
public void draw(String content material) {
System.out.println("Drawing '" + content material + "' in thick shade : " + shade);
}
}
ThinBrush.java
Code:
public class ThinBrush implements Brush {
/*
intrinsic state - shareable
*/
last BrushSize brushSize = BrushSize.THIN;
/*
extrinsic state - equipped by the shopper
*/
personal String shade = null;
public void setColor(String shade) {
this.shade = shade;
}
@Override
public void draw(String content material) {
System.out.println("Drawing ‘" + content material + "' in skinny shade : " + shade);
}
}
MediumBrush.java
Code:
public class MediumBrush implements Brush {
/*
intrinsic state - shareable
*/
last BrushSize brushSize = BrushSize.MEDIUM;
/*
extrinsic state - equipped by the shopper
*/
personal String shade = null;
public void setColor(String shade) {
this.shade = shade;
}
@Override
public void draw(String content material) {
System.out.println("Drawing '" + content material + "' in medium shade : " + shade);
}
}
Right here, the shopper will provide the extrinsic attribute of brush shade; else, the comb could have no variations. Subsequently, in essence, we are going to solely produce a sure measurement of the comb when the colour is totally different. We are going to reuse it when a unique shopper or scenario requires that brush measurement and shade.
Step 4: Subsequent, let’s Create the manufacturing unit
BrushFactory
that may present Brush
objects.
Code:
public class BrushFactory {
personal static last HashMap < String, Brush > brushMap = new HashMap < > ();
public static Brush getThickBrush(String shade) {
String key = shade + "-THICK";
Brush brush = brushMap.get(key);
if (brush != null) {
return brush;
} else {
brush = new ThickBrush();
brush.setColor(shade);
brushMap.put(key, brush);
}
return brush;
}
public static Brush getThinBrush(String shade) {
String key = shade + "-THIN";
Brush brush = brushMap.get(key);
if (brush != null) {
return brush;
} else {
brush = new ThinBrush();
brush.setColor(shade);
brushMap.put(key, brush);
}
return brush;
}
public static Brush getMediumBrush(String shade) {
String key = shade + "-MEDIUM";
Brush brush = brushMap.get(key);
if (brush != null) {
return brush;
} else {
brush = new MediumBrush();
brush.setColor(shade);
brushMap.put(key, brush);
}
return brush;
}
Step 5: Subsequent, let’s create the shopper program
FlyweightPatternDemo
.
Code:
public class FlyweightPatternDemo {
public static void major(String[] args) {
// New thick Pink Brush
Brush redThickBrush1 = BrushFactory.getThickBrush("RED");
redThickBrush1.draw("Hey There !!");
// Pink Brush is shared
Brush redThickBrush2 = BrushFactory.getThickBrush("RED");
redThickBrush2.draw("Hey There Once more !!");
System.out.println("Hashcode: " + redThickBrush1.hashCode());
System.out.println("Hashcode: " + redThickBrush2.hashCode());
// New skinny Blue Brush
Brush blueThinBrush1 = BrushFactory.getThinBrush("BLUE"); //created new pen
blueThinBrush1.draw("Hey There !!");
// Blue Brush is shared
Brush blueThinBrush2 = BrushFactory.getThinBrush("BLUE"); //created new pen
blueThinBrush2.draw("Hey There Once more!!");
System.out.println("Hashcode: " + blueThinBrush1.hashCode());
System.out.println("Hashcode: " + blueThinBrush2.hashCode());
// New MEDIUM Yellow Brush
Brush yellowThinBrush1 = BrushFactory.getMediumBrush("YELLOW"); //created new pen
yellowThinBrush1.draw("Hey There !!");
// Yellow brush is shared
Brush yellowThinBrush2 = BrushFactory.getMediumBrush("YELLOW"); //created new pen
yellowThinBrush2.draw("Hey There Once more!!");
System.out.println("Hashcode: " + yellowThinBrush1.hashCode());
System.out.println("Hashcode: " + yellowThinBrush2.hashCode());
}
}
Right here, as you possibly can see the
hashcodes
of brushes and you can find that they’re shared as they’re utilizing the identical shade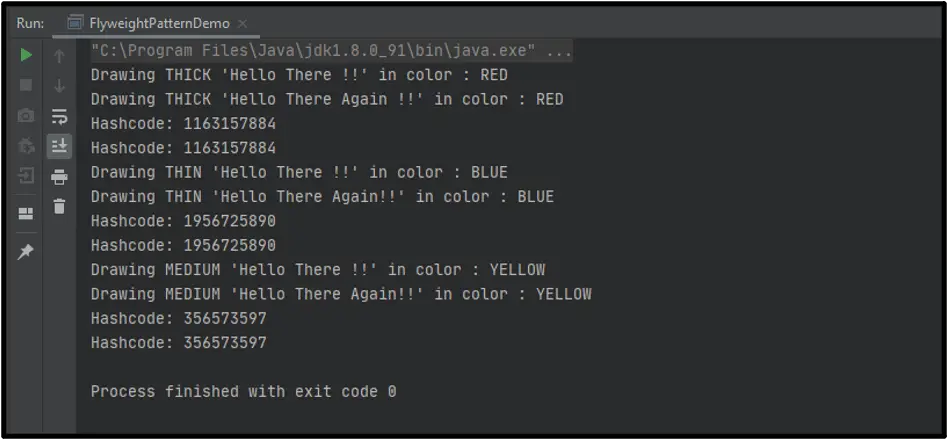
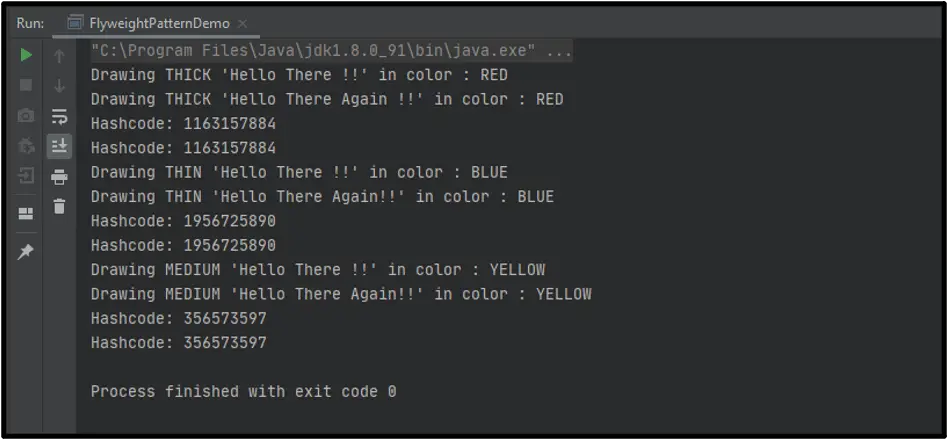
3. Class Diagram
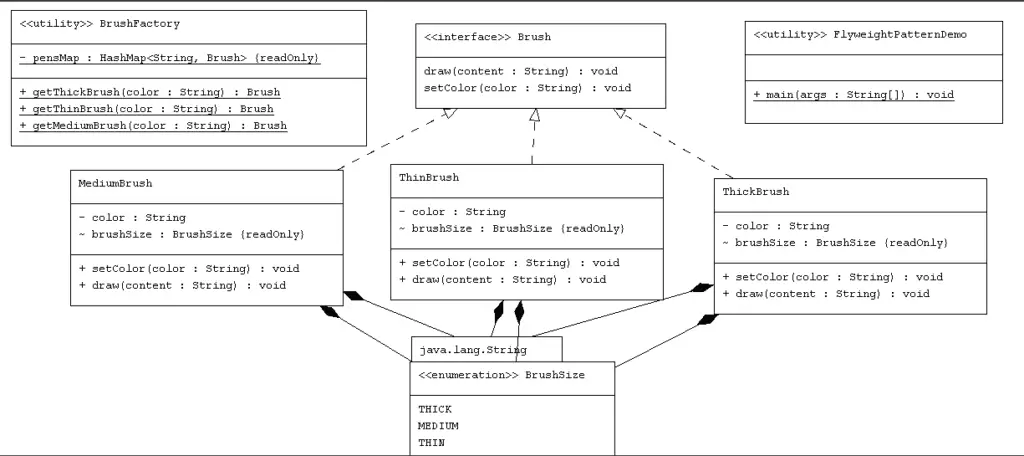
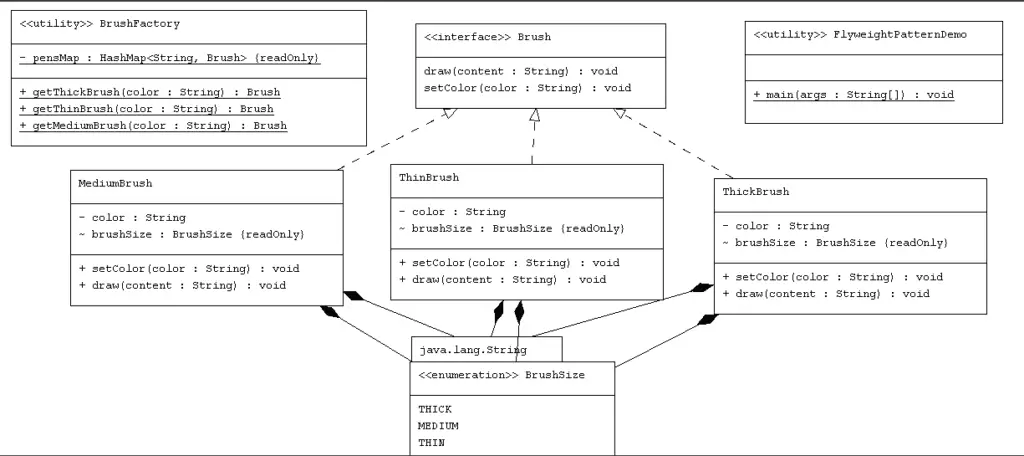
Flyweight Design Sample
3.1. Actual World Examples
Let’s check out among the actual world instance of the Flyweight Design Sample.
- Think about a brush that could be used with or and not using a refill. A brush can be utilized to provide drawings with N variety of colours as a result of a refill may be of any shade. Right here Brush could also be a flyweight with an exterior attribute equivalent to a refill. All different traits, equivalent to the comb’s physique and pointer, could also be intrinsic traits shared by all brushes. The one different solution to determine a brush is by the colour of its refill.
- The identical occasion of crimson brush can be utilized by any software modules that require entry to 1 (shared object). Solely when a unique shade brush is required will the applying module contact the flyweight manufacturing unit to order one other brush.
- One other instance of Flyweight is a string pool, the place fixed String objects shall be saved, and when a string with particular content material is required, the runtime will, if potential, return a reference to an already-existing string fixed from the pool.
- We are able to use a picture on a webpage many instances in browsers. The picture will solely be loaded as soon as by the browser; subsequent hundreds will make use of the cached model. At the moment, the identical picture is used quite a few instances. Attributable to its fastened nature and share-ability, its URL is an intrinsic attribute. Extrinsic properties, equivalent to a picture’s place coordinates, peak, and width, change relying on the situation (context) wherein they should be introduced
3.2. Flyweight Sample Benefits
- Lowers the reminiscence necessities of enormous, identically controllable objects.
- much less “full however comparable issues” are current general within the system.
- Offers a centralized technique for managing the states of quite a few “digital” objects.
3.3 Challenges
- We should give this flyweight configuration sufficient time. The preliminary value of design time and experience could also be excessive.
- We take a typical template class from the present objects and use it to make flyweights. It may be difficult to debug and keep this extra layer of code.
- The flyweight sample is steadily paired with singleton manufacturing unit implementation, and extra expense is required to guard the singularity.
4. Flyweight Sample Vs Singleton Sample
We are able to hold only one merchandise within the system due to the singleton design Sample. In different phrases, as soon as the mandatory object is produced, we’re unable to provide any extra. The present object should be used all through your complete software. When it’s vital to construct a lot of objects which can be comparable however differ primarily based on an extrinsic attribute equipped by the shopper, the flyweight sample is utilized.
Abstract
On this submit, we talked concerning the Flyweight Design Sample. We noticed the real-world examples together with what are some benefits and drawbacks of utilizing this sample. We additionally noticed a Java implementation for this design sample. You possibly can all the time examine our GitHub repository for the most recent supply code.
The ValueOf() strategies in all wrapper lessons make use of cached objects, demonstrating the applying of the Flyweight design precept. The Java String class’s implementation of the String Pool is the most effective case research.